用法
使用 v-model
指令来控制 InputMenu 的值,或使用 default-value
属性来设置不需要控制其状态时的初始值。
SelectMenu
,但它使用 Input 而非 Select。项目
将 items
属性用作字符串、数字或布尔值的数组。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" :items="items" />
</template>
您也可以传入包含以下属性的对象数组:
label?: string
type?: "label" | "separator" | "item"
icon?: string
avatar?: AvatarProps
chip?: ChipProps
disabled?: boolean
onSelect?(e: Event): void
<script setup lang="ts">
const items = ref([
{
label: 'Backlog'
},
{
label: 'Todo'
},
{
label: 'In Progress'
},
{
label: 'Done'
}
])
const value = ref({
label: 'Todo'
})
</script>
<template>
<UInputMenu v-model="value" :items="items" />
</template>
您也可以向 items
属性传入数组的数组,以显示分组的项目。
<script setup lang="ts">
const items = ref([
['Apple', 'Banana', 'Blueberry', 'Grapes', 'Pineapple'],
['Aubergine', 'Broccoli', 'Carrot', 'Courgette', 'Leek']
])
const value = ref('Apple')
</script>
<template>
<UInputMenu v-model="value" :items="items" />
</template>
值键
您可以通过使用 value-key
属性来绑定对象的单个属性,而不是整个对象。默认为 undefined
。
<script setup lang="ts">
const items = ref([
{
label: 'Backlog',
id: 'backlog'
},
{
label: 'Todo',
id: 'todo'
},
{
label: 'In Progress',
id: 'in_progress'
},
{
label: 'Done',
id: 'done'
}
])
const value = ref('todo')
</script>
<template>
<UInputMenu v-model="value" value-key="id" :items="items" />
</template>
多选
使用 multiple
属性以允许多项选择,选中的项目将显示为徽章。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref(['Backlog', 'Todo'])
</script>
<template>
<UInputMenu v-model="value" multiple :items="items" />
</template>
default-value
属性或 v-model
指令传入一个数组。删除图标
结合 multiple
使用时,可使用 delete-icon
属性自定义徽章中的删除 Icon。默认为 i-lucide-x
。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref(['Backlog', 'Todo'])
</script>
<template>
<UInputMenu v-model="value" multiple delete-icon="i-lucide-trash" :items="items" />
</template>
占位符
使用 placeholder
属性设置占位文本。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
</script>
<template>
<UInputMenu placeholder="Select status" :items="items" />
</template>
内容
使用 content
属性控制 InputMenu 内容如何渲染,例如其 align
或 side
。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu
v-model="value"
:content="{
align: 'center',
side: 'bottom',
sideOffset: 8
}"
:items="items"
/>
</template>
箭头
使用 arrow
属性在 InputMenu 上显示箭头。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" arrow :items="items" />
</template>
颜色
使用 color
属性更改 InputMenu 聚焦时的环形颜色。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" color="neutral" highlight :items="items" />
</template>
highlight
属性来显示聚焦状态。当发生验证错误时,它在内部使用。变体
使用 variant
属性更改 InputMenu 的变体。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" color="neutral" variant="subtle" :items="items" />
</template>
尺寸
使用 size
属性更改 InputMenu 的尺寸。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" size="xl" :items="items" />
</template>
Icon
使用 icon
属性在 InputMenu 内显示一个 Icon。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" icon="i-lucide-search" size="md" :items="items" />
</template>
尾部图标
使用 trailing-icon
属性自定义尾部 Icon。默认为 i-lucide-chevron-down
。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" trailing-icon="i-lucide-arrow-down" size="md" :items="items" />
</template>
选中图标
使用 selected-icon
属性自定义选中项目时的图标。默认为 i-lucide-check
。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" selected-icon="i-lucide-flame" size="md" :items="items" />
</template>
Avatar
使用 avatar
属性在 InputMenu 内显示一个 Avatar。
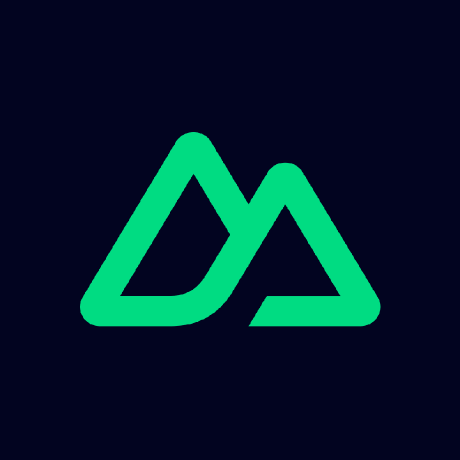
<script setup lang="ts">
const items = ref(['Nuxt', 'NuxtHub', 'NuxtLabs', 'Nuxt Modules', 'Nuxt Community'])
const value = ref('Nuxt')
</script>
<template>
<UInputMenu
v-model="value"
:avatar="{
src: 'https://github.com/nuxt.png'
}"
:items="items"
/>
</template>
加载
使用 loading
属性在 InputMenu 上显示加载图标。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" loading :items="items" />
</template>
加载图标
使用 loading-icon
属性自定义加载图标。默认为 i-lucide-refresh-cw
。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" loading loading-icon="i-lucide-repeat-2" :items="items" />
</template>
禁用
使用 disabled
属性禁用 InputMenu。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
</script>
<template>
<UInputMenu disabled placeholder="Select status" :items="items" />
</template>
示例
使用 items 类型
您可以使用带有 separator
的 type
属性在项目之间显示分隔符,或使用 label
显示标签。
<script setup lang="ts">
const items = ref([
{
type: 'label',
label: 'Fruits'
},
'Apple',
'Banana',
'Blueberry',
'Grapes',
'Pineapple',
{
type: 'separator'
},
{
type: 'label',
label: 'Vegetables'
},
'Aubergine',
'Broccoli',
'Carrot',
'Courgette',
'Leek'
])
const value = ref('Apple')
</script>
<template>
<UInputMenu v-model="value" :items="items" />
</template>
项目中包含图标
您可以使用 icon
属性在项目内显示一个 Icon。
<script setup lang="ts">
import type { InputMenuItem } from '@nuxt/ui'
const items = ref([
{
label: 'Backlog',
value: 'backlog',
icon: 'i-lucide-circle-help'
},
{
label: 'Todo',
value: 'todo',
icon: 'i-lucide-circle-plus'
},
{
label: 'In Progress',
value: 'in_progress',
icon: 'i-lucide-circle-arrow-up'
},
{
label: 'Done',
value: 'done',
icon: 'i-lucide-circle-check'
}
] satisfies InputMenuItem[])
const value = ref(items.value[0])
</script>
<template>
<UInputMenu v-model="value" :icon="value?.icon" :items="items" />
</template>
#leading
插槽显示选中的图标。项目中包含头像
您可以使用 avatar
属性在项目内显示一个 Avatar。
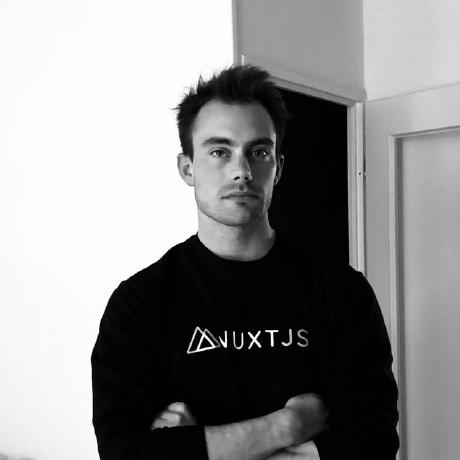
<script setup lang="ts">
import type { InputMenuItem } from '@nuxt/ui'
const items = ref([
{
label: 'benjamincanac',
value: 'benjamincanac',
avatar: {
src: 'https://github.com/benjamincanac.png',
alt: 'benjamincanac'
}
},
{
label: 'romhml',
value: 'romhml',
avatar: {
src: 'https://github.com/romhml.png',
alt: 'romhml'
}
},
{
label: 'noook',
value: 'noook',
avatar: {
src: 'https://github.com/noook.png',
alt: 'noook'
}
},
{
label: 'sandros94',
value: 'sandros94',
avatar: {
src: 'https://github.com/sandros94.png',
alt: 'sandros94'
}
}
] satisfies InputMenuItem[])
const value = ref(items.value[0])
</script>
<template>
<UInputMenu v-model="value" :avatar="value?.avatar" :items="items" />
</template>
#leading
插槽显示选中的头像。项目中包含 Chip
您可以使用 chip
属性在项目内显示一个 Chip。
<script setup lang="ts">
import type { InputMenuItem, ChipProps } from '@nuxt/ui'
const items = ref([
{
label: 'bug',
value: 'bug',
chip: {
color: 'error'
}
},
{
label: 'feature',
value: 'feature',
chip: {
color: 'success'
}
},
{
label: 'enhancement',
value: 'enhancement',
chip: {
color: 'info'
}
}
] satisfies InputMenuItem[])
const value = ref(items.value[0])
</script>
<template>
<UInputMenu v-model="value" :items="items">
<template #leading="{ modelValue, ui }">
<UChip
v-if="modelValue"
v-bind="modelValue.chip"
inset
standalone
:size="(ui.itemLeadingChipSize() as ChipProps['size'])"
:class="ui.itemLeadingChip()"
/>
</template>
</UInputMenu>
</template>
#leading
插槽显示选中的 Chip。控制打开状态
您可以使用 default-open
属性或 v-model:open
指令来控制打开状态。
<script setup lang="ts">
const open = ref(false)
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
defineShortcuts({
o: () => open.value = !open.value
})
</script>
<template>
<UInputMenu v-model="value" v-model:open="open" :items="items" />
</template>
defineShortcuts
,您可以通过按 O 键切换 InputMenu。控制聚焦时的打开状态
您也可以使用 @focus
指令控制打开状态。
<script setup lang="ts">
const open = ref(false)
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const selected = ref('Backlog')
</script>
<template>
<UInputMenu
v-model="selected"
v-model:open="open"
:items="items"
@focus="open = true"
/>
</template>
控制搜索词
使用 v-model:search-term
指令控制搜索词。
<script setup lang="ts">
const searchTerm = ref('D')
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu v-model="value" v-model:search-term="searchTerm" :items="items" />
</template>
带有旋转图标
这是一个带有旋转图标的示例,该图标指示 InputMenu 的打开状态。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
</script>
<template>
<UInputMenu
v-model="value"
:items="items"
:ui="{
trailingIcon: 'group-data-[state=open]:rotate-180 transition-transform duration-200'
}"
/>
</template>
带有创建项目功能
使用 create-item
属性使用户能够添加不在预定义选项中的自定义值。
<script setup lang="ts">
const items = ref(['Backlog', 'Todo', 'In Progress', 'Done'])
const value = ref('Backlog')
function onCreate(item: string) {
items.value.push(item)
value.value = item
}
</script>
<template>
<UInputMenu
v-model="value"
create-item
:items="items"
class="w-48"
@create="onCreate"
/>
</template>
always
可即使存在相似值时也显示该选项。使用获取的项目
您可以从 API 获取项目并在 InputMenu 中使用。
<script setup lang="ts">
import type { AvatarProps } from '@nuxt/ui'
const { data: users, status } = await useFetch('https://jsonplaceholder.typicode.com/users', {
key: 'typicode-users',
transform: (data: { id: number, name: string }[]) => {
return data?.map(user => ({
label: user.name,
value: String(user.id),
avatar: { src: `https://i.pravatar.cc/120?img=${user.id}` }
}))
},
lazy: true
})
</script>
<template>
<UInputMenu
:items="users"
:loading="status === 'pending'"
icon="i-lucide-user"
placeholder="Select user"
>
<template #leading="{ modelValue, ui }">
<UAvatar
v-if="modelValue"
v-bind="modelValue.avatar"
:size="(ui.leadingAvatarSize() as AvatarProps['size'])"
:class="ui.leadingAvatar()"
/>
</template>
</UInputMenu>
</template>
带有忽略过滤器功能
将 ignore-filter
属性设置为 true
可禁用内部搜索并使用您自己的搜索逻辑。
<script setup lang="ts">
import type { AvatarProps } from '@nuxt/ui'
const searchTerm = ref('')
const searchTermDebounced = refDebounced(searchTerm, 200)
const { data: users, status } = await useFetch('https://jsonplaceholder.typicode.com/users', {
key: 'typicode-users',
params: { q: searchTermDebounced },
transform: (data: { id: number, name: string }[]) => {
return data?.map(user => ({
label: user.name,
value: String(user.id),
avatar: { src: `https://i.pravatar.cc/120?img=${user.id}` }
}))
},
lazy: true
})
</script>
<template>
<UInputMenu
v-model:search-term="searchTerm"
:items="users"
:loading="status === 'pending'"
ignore-filter
icon="i-lucide-user"
placeholder="Select user"
>
<template #leading="{ modelValue, ui }">
<UAvatar
v-if="modelValue"
v-bind="modelValue.avatar"
:size="(ui.leadingAvatarSize() as AvatarProps['size'])"
:class="ui.leadingAvatar()"
/>
</template>
</UInputMenu>
</template>
refDebounced
来 debounce (防抖) API 调用。带有过滤字段功能
使用 filter-fields
属性和一个字段数组来指定过滤字段。默认为 [labelKey]
。
<script setup lang="ts">
import type { AvatarProps } from '@nuxt/ui'
const { data: users, status } = await useFetch('https://jsonplaceholder.typicode.com/users', {
key: 'typicode-users-email',
transform: (data: { id: number, name: string, email: string }[]) => {
return data?.map(user => ({
label: user.name,
email: user.email,
value: String(user.id),
avatar: { src: `https://i.pravatar.cc/120?img=${user.id}` }
}))
},
lazy: true
})
</script>
<template>
<UInputMenu
:items="users"
:loading="status === 'pending'"
:filter-fields="['label', 'email']"
icon="i-lucide-user"
placeholder="Select user"
class="w-80"
>
<template #leading="{ modelValue, ui }">
<UAvatar
v-if="modelValue"
v-bind="modelValue.avatar"
:size="(ui.leadingAvatarSize() as AvatarProps['size'])"
:class="ui.leadingAvatar()"
/>
</template>
<template #item-label="{ item }">
{{ item.label }}
<span class="text-muted">
{{ item.email }}
</span>
</template>
</UInputMenu>
</template>
作为国家/地区选择器
此示例演示了如何将 InputMenu 用作具有懒加载功能的国家/地区选择器 - 国家/地区仅在菜单打开时获取。
<script setup lang="ts">
const { data: countries, status, execute } = await useLazyFetch<{
name: string
code: string
emoji: string
}[]>('/api/countries.json', {
immediate: false
})
function onOpen() {
if (!countries.value?.length) {
execute()
}
}
</script>
<template>
<UInputMenu
:items="countries"
:loading="status === 'pending'"
label-key="name"
:search-input="{ icon: 'i-lucide-search' }"
placeholder="Select country"
class="w-48"
@update:open="onOpen"
>
<template #leading="{ modelValue, ui }">
<span v-if="modelValue" class="size-5 text-center">
{{ modelValue?.emoji }}
</span>
<UIcon v-else name="i-lucide-earth" :class="ui.leadingIcon()" />
</template>
<template #item-leading="{ item }">
<span class="size-5 text-center">
{{ item.emoji }}
</span>
</template>
</UInputMenu>
</template>
API
属性
属性 | 默认值 | 类型 |
---|---|---|
as |
|
该组件应渲染为的元素或组件。 |
id |
| |
type |
|
|
placeholder |
输入为空时的占位文本。 | |
color |
|
|
variant |
|
|
size |
|
|
必填项 |
| |
自动聚焦 |
| |
自动聚焦延迟 |
|
|
trailingIcon |
|
用于打开菜单时显示的图标。 |
selectedIcon |
|
选中项时显示的图标。 |
deleteIcon |
|
用于删除标签时显示的图标。仅当 |
内容 |
|
菜单的内容。
|
箭头 |
|
在菜单旁边显示一个箭头。 |
Portal |
|
在 Portal 中渲染菜单。 |
值键 |
|
当 |
标签键 |
|
当 |
项目 |
| |
默认值 |
InputMenu 初次渲染时的值。当您不需要控制 InputMenu 的状态时使用。 | |
模型值 |
InputMenu 的受控值。可以使用 | |
多选 |
是否可以选中多个选项。 | |
highlight |
高亮显示环形颜色,如同焦点状态。 | |
创建项目 |
|
确定是否可以添加选项中不存在的自定义用户输入。
|
过滤字段 |
|
用于过滤项目的字段。 |
忽略过滤 |
|
当 |
打开 |
Combobox 的受控打开状态。可以使用 | |
默认打开状态 |
Combobox 初次渲染时的打开状态。 | |
disabled |
当 | |
名称 |
字段名称。与其所属表单一起作为名称/值对提交。 | |
resetSearchTermOnBlur |
|
Combobox 输入框失焦时是否重置 searchTerm |
resetSearchTermOnSelect |
|
Combobox 值被选中时是否重置 searchTerm |
highlightOnHover |
当 | |
icon |
根据 | |
avatar |
在左侧显示头像。
| |
leading |
当 | |
leadingIcon |
在左侧显示一个图标。 | |
trailing |
当 | |
loading |
当 | |
loadingIcon |
|
当 |
searchTerm |
|
|
ui |
|
插槽
插槽 | 类型 |
---|---|
leading |
|
trailing |
|
empty |
|
item |
|
item-leading |
|
item-label |
|
item-trailing |
|
tags-item-text |
|
tags-item-delete |
|
content-top |
|
content-bottom |
|
create-item-label |
|
触发事件
事件 | 类型 |
---|---|
blur |
|
change |
|
focus |
|
update:open |
|
create |
|
highlight |
|
update:modelValue |
|
update:searchTerm |
|
主题
export default defineAppConfig({
ui: {
inputMenu: {
slots: {
root: 'relative inline-flex items-center',
base: [
'rounded-md',
'transition-colors'
],
leading: 'absolute inset-y-0 start-0 flex items-center',
leadingIcon: 'shrink-0 text-dimmed',
leadingAvatar: 'shrink-0',
leadingAvatarSize: '',
trailing: 'group absolute inset-y-0 end-0 flex items-center disabled:cursor-not-allowed disabled:opacity-75',
trailingIcon: 'shrink-0 text-dimmed',
arrow: 'fill-default',
content: 'max-h-60 w-(--reka-combobox-trigger-width) bg-default shadow-lg rounded-md ring ring-default overflow-hidden data-[state=open]:animate-[scale-in_100ms_ease-out] data-[state=closed]:animate-[scale-out_100ms_ease-in] origin-(--reka-combobox-content-transform-origin) pointer-events-auto',
viewport: 'divide-y divide-default scroll-py-1',
group: 'p-1 isolate',
empty: 'py-2 text-center text-sm text-muted',
label: 'font-semibold text-highlighted',
separator: '-mx-1 my-1 h-px bg-border',
item: [
'group relative w-full flex items-center gap-1.5 p-1.5 text-sm select-none outline-none before:absolute before:z-[-1] before:inset-px before:rounded-md data-disabled:cursor-not-allowed data-disabled:opacity-75 text-default data-highlighted:not-data-disabled:text-highlighted data-highlighted:not-data-disabled:before:bg-elevated/50',
'transition-colors before:transition-colors'
],
itemLeadingIcon: [
'shrink-0 text-dimmed group-data-highlighted:not-group-data-disabled:text-default',
'transition-colors'
],
itemLeadingAvatar: 'shrink-0',
itemLeadingAvatarSize: '',
itemLeadingChip: 'shrink-0',
itemLeadingChipSize: '',
itemTrailing: 'ms-auto inline-flex gap-1.5 items-center',
itemTrailingIcon: 'shrink-0',
itemLabel: 'truncate',
tagsItem: 'px-1.5 py-0.5 rounded-sm font-medium inline-flex items-center gap-0.5 ring ring-inset ring-accented bg-elevated text-default data-disabled:cursor-not-allowed data-disabled:opacity-75',
tagsItemText: 'truncate',
tagsItemDelete: [
'inline-flex items-center rounded-xs text-dimmed hover:text-default hover:bg-accented/75 disabled:pointer-events-none',
'transition-colors'
],
tagsItemDeleteIcon: '',
tagsInput: ''
},
variants: {
buttonGroup: {
horizontal: {
root: 'group',
base: 'group-not-only:group-first:rounded-e-none group-not-only:group-last:rounded-s-none group-not-last:group-not-first:rounded-none'
},
vertical: {
root: 'group',
base: 'group-not-only:group-first:rounded-b-none group-not-only:group-last:rounded-t-none group-not-last:group-not-first:rounded-none'
}
},
size: {
xs: {
base: 'px-2 py-1 text-xs gap-1',
leading: 'ps-2',
trailing: 'pe-2',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4',
label: 'p-1 text-[10px]/3 gap-1',
item: 'p-1 text-xs gap-1',
itemLeadingIcon: 'size-4',
itemLeadingAvatarSize: '3xs',
itemLeadingChip: 'size-4',
itemLeadingChipSize: 'sm',
itemTrailingIcon: 'size-4',
tagsItem: 'text-[10px]/3',
tagsItemDeleteIcon: 'size-3'
},
sm: {
base: 'px-2.5 py-1.5 text-xs gap-1.5',
leading: 'ps-2.5',
trailing: 'pe-2.5',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4',
label: 'p-1.5 text-[10px]/3 gap-1.5',
item: 'p-1.5 text-xs gap-1.5',
itemLeadingIcon: 'size-4',
itemLeadingAvatarSize: '3xs',
itemLeadingChip: 'size-4',
itemLeadingChipSize: 'sm',
itemTrailingIcon: 'size-4',
tagsItem: 'text-[10px]/3',
tagsItemDeleteIcon: 'size-3'
},
md: {
base: 'px-2.5 py-1.5 text-sm gap-1.5',
leading: 'ps-2.5',
trailing: 'pe-2.5',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5',
label: 'p-1.5 text-xs gap-1.5',
item: 'p-1.5 text-sm gap-1.5',
itemLeadingIcon: 'size-5',
itemLeadingAvatarSize: '2xs',
itemLeadingChip: 'size-5',
itemLeadingChipSize: 'md',
itemTrailingIcon: 'size-5',
tagsItem: 'text-xs',
tagsItemDeleteIcon: 'size-3.5'
},
lg: {
base: 'px-3 py-2 text-sm gap-2',
leading: 'ps-3',
trailing: 'pe-3',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5',
label: 'p-2 text-xs gap-2',
item: 'p-2 text-sm gap-2',
itemLeadingIcon: 'size-5',
itemLeadingAvatarSize: '2xs',
itemLeadingChip: 'size-5',
itemLeadingChipSize: 'md',
itemTrailingIcon: 'size-5',
tagsItem: 'text-xs',
tagsItemDeleteIcon: 'size-3.5'
},
xl: {
base: 'px-3 py-2 text-base gap-2',
leading: 'ps-3',
trailing: 'pe-3',
leadingIcon: 'size-6',
leadingAvatarSize: 'xs',
trailingIcon: 'size-6',
label: 'p-2 text-sm gap-2',
item: 'p-2 text-base gap-2',
itemLeadingIcon: 'size-6',
itemLeadingAvatarSize: 'xs',
itemLeadingChip: 'size-6',
itemLeadingChipSize: 'lg',
itemTrailingIcon: 'size-6',
tagsItem: 'text-sm',
tagsItemDeleteIcon: 'size-4'
}
},
variant: {
outline: 'text-highlighted bg-default ring ring-inset ring-accented',
soft: 'text-highlighted bg-elevated/50 hover:bg-elevated focus:bg-elevated disabled:bg-elevated/50',
subtle: 'text-highlighted bg-elevated ring ring-inset ring-accented',
ghost: 'text-highlighted bg-transparent hover:bg-elevated focus:bg-elevated disabled:bg-transparent dark:disabled:bg-transparent',
none: 'text-highlighted bg-transparent'
},
color: {
primary: '',
secondary: '',
success: '',
info: '',
warning: '',
error: '',
neutral: ''
},
leading: {
true: ''
},
trailing: {
true: ''
},
loading: {
true: ''
},
highlight: {
true: ''
},
type: {
file: 'file:me-1.5 file:font-medium file:text-muted file:outline-none'
},
multiple: {
true: {
root: 'flex-wrap',
tagsInput: 'border-0 bg-transparent placeholder:text-dimmed focus:outline-none disabled:cursor-not-allowed disabled:opacity-75'
},
false: {
base: 'w-full border-0 placeholder:text-dimmed focus:outline-none disabled:cursor-not-allowed disabled:opacity-75'
}
}
},
compoundVariants: [
{
color: 'primary',
multiple: true,
variant: [
'outline',
'subtle'
],
class: 'has-focus-visible:ring-2 has-focus-visible:ring-primary'
},
{
color: 'neutral',
multiple: true,
variant: [
'outline',
'subtle'
],
class: 'has-focus-visible:ring-2 has-focus-visible:ring-inverted'
},
{
color: 'primary',
variant: [
'outline',
'subtle'
],
class: 'focus-visible:ring-2 focus-visible:ring-inset focus-visible:ring-primary'
},
{
color: 'primary',
highlight: true,
class: 'ring ring-inset ring-primary'
},
{
color: 'neutral',
variant: [
'outline',
'subtle'
],
class: 'focus-visible:ring-2 focus-visible:ring-inset focus-visible:ring-inverted'
},
{
color: 'neutral',
highlight: true,
class: 'ring ring-inset ring-inverted'
},
{
leading: true,
size: 'xs',
class: 'ps-7'
},
{
leading: true,
size: 'sm',
class: 'ps-8'
},
{
leading: true,
size: 'md',
class: 'ps-9'
},
{
leading: true,
size: 'lg',
class: 'ps-10'
},
{
leading: true,
size: 'xl',
class: 'ps-11'
},
{
trailing: true,
size: 'xs',
class: 'pe-7'
},
{
trailing: true,
size: 'sm',
class: 'pe-8'
},
{
trailing: true,
size: 'md',
class: 'pe-9'
},
{
trailing: true,
size: 'lg',
class: 'pe-10'
},
{
trailing: true,
size: 'xl',
class: 'pe-11'
},
{
loading: true,
leading: true,
class: {
leadingIcon: 'animate-spin'
}
},
{
loading: true,
leading: false,
trailing: true,
class: {
trailingIcon: 'animate-spin'
}
}
],
defaultVariants: {
size: 'md',
color: 'primary',
variant: 'outline'
}
}
}
})
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import ui from '@nuxt/ui/vite'
export default defineConfig({
plugins: [
vue(),
ui({
ui: {
inputMenu: {
slots: {
root: 'relative inline-flex items-center',
base: [
'rounded-md',
'transition-colors'
],
leading: 'absolute inset-y-0 start-0 flex items-center',
leadingIcon: 'shrink-0 text-dimmed',
leadingAvatar: 'shrink-0',
leadingAvatarSize: '',
trailing: 'group absolute inset-y-0 end-0 flex items-center disabled:cursor-not-allowed disabled:opacity-75',
trailingIcon: 'shrink-0 text-dimmed',
arrow: 'fill-default',
content: 'max-h-60 w-(--reka-combobox-trigger-width) bg-default shadow-lg rounded-md ring ring-default overflow-hidden data-[state=open]:animate-[scale-in_100ms_ease-out] data-[state=closed]:animate-[scale-out_100ms_ease-in] origin-(--reka-combobox-content-transform-origin) pointer-events-auto',
viewport: 'divide-y divide-default scroll-py-1',
group: 'p-1 isolate',
empty: 'py-2 text-center text-sm text-muted',
label: 'font-semibold text-highlighted',
separator: '-mx-1 my-1 h-px bg-border',
item: [
'group relative w-full flex items-center gap-1.5 p-1.5 text-sm select-none outline-none before:absolute before:z-[-1] before:inset-px before:rounded-md data-disabled:cursor-not-allowed data-disabled:opacity-75 text-default data-highlighted:not-data-disabled:text-highlighted data-highlighted:not-data-disabled:before:bg-elevated/50',
'transition-colors before:transition-colors'
],
itemLeadingIcon: [
'shrink-0 text-dimmed group-data-highlighted:not-group-data-disabled:text-default',
'transition-colors'
],
itemLeadingAvatar: 'shrink-0',
itemLeadingAvatarSize: '',
itemLeadingChip: 'shrink-0',
itemLeadingChipSize: '',
itemTrailing: 'ms-auto inline-flex gap-1.5 items-center',
itemTrailingIcon: 'shrink-0',
itemLabel: 'truncate',
tagsItem: 'px-1.5 py-0.5 rounded-sm font-medium inline-flex items-center gap-0.5 ring ring-inset ring-accented bg-elevated text-default data-disabled:cursor-not-allowed data-disabled:opacity-75',
tagsItemText: 'truncate',
tagsItemDelete: [
'inline-flex items-center rounded-xs text-dimmed hover:text-default hover:bg-accented/75 disabled:pointer-events-none',
'transition-colors'
],
tagsItemDeleteIcon: '',
tagsInput: ''
},
variants: {
buttonGroup: {
horizontal: {
root: 'group',
base: 'group-not-only:group-first:rounded-e-none group-not-only:group-last:rounded-s-none group-not-last:group-not-first:rounded-none'
},
vertical: {
root: 'group',
base: 'group-not-only:group-first:rounded-b-none group-not-only:group-last:rounded-t-none group-not-last:group-not-first:rounded-none'
}
},
size: {
xs: {
base: 'px-2 py-1 text-xs gap-1',
leading: 'ps-2',
trailing: 'pe-2',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4',
label: 'p-1 text-[10px]/3 gap-1',
item: 'p-1 text-xs gap-1',
itemLeadingIcon: 'size-4',
itemLeadingAvatarSize: '3xs',
itemLeadingChip: 'size-4',
itemLeadingChipSize: 'sm',
itemTrailingIcon: 'size-4',
tagsItem: 'text-[10px]/3',
tagsItemDeleteIcon: 'size-3'
},
sm: {
base: 'px-2.5 py-1.5 text-xs gap-1.5',
leading: 'ps-2.5',
trailing: 'pe-2.5',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4',
label: 'p-1.5 text-[10px]/3 gap-1.5',
item: 'p-1.5 text-xs gap-1.5',
itemLeadingIcon: 'size-4',
itemLeadingAvatarSize: '3xs',
itemLeadingChip: 'size-4',
itemLeadingChipSize: 'sm',
itemTrailingIcon: 'size-4',
tagsItem: 'text-[10px]/3',
tagsItemDeleteIcon: 'size-3'
},
md: {
base: 'px-2.5 py-1.5 text-sm gap-1.5',
leading: 'ps-2.5',
trailing: 'pe-2.5',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5',
label: 'p-1.5 text-xs gap-1.5',
item: 'p-1.5 text-sm gap-1.5',
itemLeadingIcon: 'size-5',
itemLeadingAvatarSize: '2xs',
itemLeadingChip: 'size-5',
itemLeadingChipSize: 'md',
itemTrailingIcon: 'size-5',
tagsItem: 'text-xs',
tagsItemDeleteIcon: 'size-3.5'
},
lg: {
base: 'px-3 py-2 text-sm gap-2',
leading: 'ps-3',
trailing: 'pe-3',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5',
label: 'p-2 text-xs gap-2',
item: 'p-2 text-sm gap-2',
itemLeadingIcon: 'size-5',
itemLeadingAvatarSize: '2xs',
itemLeadingChip: 'size-5',
itemLeadingChipSize: 'md',
itemTrailingIcon: 'size-5',
tagsItem: 'text-xs',
tagsItemDeleteIcon: 'size-3.5'
},
xl: {
base: 'px-3 py-2 text-base gap-2',
leading: 'ps-3',
trailing: 'pe-3',
leadingIcon: 'size-6',
leadingAvatarSize: 'xs',
trailingIcon: 'size-6',
label: 'p-2 text-sm gap-2',
item: 'p-2 text-base gap-2',
itemLeadingIcon: 'size-6',
itemLeadingAvatarSize: 'xs',
itemLeadingChip: 'size-6',
itemLeadingChipSize: 'lg',
itemTrailingIcon: 'size-6',
tagsItem: 'text-sm',
tagsItemDeleteIcon: 'size-4'
}
},
variant: {
outline: 'text-highlighted bg-default ring ring-inset ring-accented',
soft: 'text-highlighted bg-elevated/50 hover:bg-elevated focus:bg-elevated disabled:bg-elevated/50',
subtle: 'text-highlighted bg-elevated ring ring-inset ring-accented',
ghost: 'text-highlighted bg-transparent hover:bg-elevated focus:bg-elevated disabled:bg-transparent dark:disabled:bg-transparent',
none: 'text-highlighted bg-transparent'
},
color: {
primary: '',
secondary: '',
success: '',
info: '',
warning: '',
error: '',
neutral: ''
},
leading: {
true: ''
},
trailing: {
true: ''
},
loading: {
true: ''
},
highlight: {
true: ''
},
type: {
file: 'file:me-1.5 file:font-medium file:text-muted file:outline-none'
},
multiple: {
true: {
root: 'flex-wrap',
tagsInput: 'border-0 bg-transparent placeholder:text-dimmed focus:outline-none disabled:cursor-not-allowed disabled:opacity-75'
},
false: {
base: 'w-full border-0 placeholder:text-dimmed focus:outline-none disabled:cursor-not-allowed disabled:opacity-75'
}
}
},
compoundVariants: [
{
color: 'primary',
multiple: true,
variant: [
'outline',
'subtle'
],
class: 'has-focus-visible:ring-2 has-focus-visible:ring-primary'
},
{
color: 'neutral',
multiple: true,
variant: [
'outline',
'subtle'
],
class: 'has-focus-visible:ring-2 has-focus-visible:ring-inverted'
},
{
color: 'primary',
variant: [
'outline',
'subtle'
],
class: 'focus-visible:ring-2 focus-visible:ring-inset focus-visible:ring-primary'
},
{
color: 'primary',
highlight: true,
class: 'ring ring-inset ring-primary'
},
{
color: 'neutral',
variant: [
'outline',
'subtle'
],
class: 'focus-visible:ring-2 focus-visible:ring-inset focus-visible:ring-inverted'
},
{
color: 'neutral',
highlight: true,
class: 'ring ring-inset ring-inverted'
},
{
leading: true,
size: 'xs',
class: 'ps-7'
},
{
leading: true,
size: 'sm',
class: 'ps-8'
},
{
leading: true,
size: 'md',
class: 'ps-9'
},
{
leading: true,
size: 'lg',
class: 'ps-10'
},
{
leading: true,
size: 'xl',
class: 'ps-11'
},
{
trailing: true,
size: 'xs',
class: 'pe-7'
},
{
trailing: true,
size: 'sm',
class: 'pe-8'
},
{
trailing: true,
size: 'md',
class: 'pe-9'
},
{
trailing: true,
size: 'lg',
class: 'pe-10'
},
{
trailing: true,
size: 'xl',
class: 'pe-11'
},
{
loading: true,
leading: true,
class: {
leadingIcon: 'animate-spin'
}
},
{
loading: true,
leading: false,
trailing: true,
class: {
trailingIcon: 'animate-spin'
}
}
],
defaultVariants: {
size: 'md',
color: 'primary',
variant: 'outline'
}
}
}
})
]
})
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import uiPro from '@nuxt/ui-pro/vite'
export default defineConfig({
plugins: [
vue(),
uiPro({
ui: {
inputMenu: {
slots: {
root: 'relative inline-flex items-center',
base: [
'rounded-md',
'transition-colors'
],
leading: 'absolute inset-y-0 start-0 flex items-center',
leadingIcon: 'shrink-0 text-dimmed',
leadingAvatar: 'shrink-0',
leadingAvatarSize: '',
trailing: 'group absolute inset-y-0 end-0 flex items-center disabled:cursor-not-allowed disabled:opacity-75',
trailingIcon: 'shrink-0 text-dimmed',
arrow: 'fill-default',
content: 'max-h-60 w-(--reka-combobox-trigger-width) bg-default shadow-lg rounded-md ring ring-default overflow-hidden data-[state=open]:animate-[scale-in_100ms_ease-out] data-[state=closed]:animate-[scale-out_100ms_ease-in] origin-(--reka-combobox-content-transform-origin) pointer-events-auto',
viewport: 'divide-y divide-default scroll-py-1',
group: 'p-1 isolate',
empty: 'py-2 text-center text-sm text-muted',
label: 'font-semibold text-highlighted',
separator: '-mx-1 my-1 h-px bg-border',
item: [
'group relative w-full flex items-center gap-1.5 p-1.5 text-sm select-none outline-none before:absolute before:z-[-1] before:inset-px before:rounded-md data-disabled:cursor-not-allowed data-disabled:opacity-75 text-default data-highlighted:not-data-disabled:text-highlighted data-highlighted:not-data-disabled:before:bg-elevated/50',
'transition-colors before:transition-colors'
],
itemLeadingIcon: [
'shrink-0 text-dimmed group-data-highlighted:not-group-data-disabled:text-default',
'transition-colors'
],
itemLeadingAvatar: 'shrink-0',
itemLeadingAvatarSize: '',
itemLeadingChip: 'shrink-0',
itemLeadingChipSize: '',
itemTrailing: 'ms-auto inline-flex gap-1.5 items-center',
itemTrailingIcon: 'shrink-0',
itemLabel: 'truncate',
tagsItem: 'px-1.5 py-0.5 rounded-sm font-medium inline-flex items-center gap-0.5 ring ring-inset ring-accented bg-elevated text-default data-disabled:cursor-not-allowed data-disabled:opacity-75',
tagsItemText: 'truncate',
tagsItemDelete: [
'inline-flex items-center rounded-xs text-dimmed hover:text-default hover:bg-accented/75 disabled:pointer-events-none',
'transition-colors'
],
tagsItemDeleteIcon: '',
tagsInput: ''
},
variants: {
buttonGroup: {
horizontal: {
root: 'group',
base: 'group-not-only:group-first:rounded-e-none group-not-only:group-last:rounded-s-none group-not-last:group-not-first:rounded-none'
},
vertical: {
root: 'group',
base: 'group-not-only:group-first:rounded-b-none group-not-only:group-last:rounded-t-none group-not-last:group-not-first:rounded-none'
}
},
size: {
xs: {
base: 'px-2 py-1 text-xs gap-1',
leading: 'ps-2',
trailing: 'pe-2',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4',
label: 'p-1 text-[10px]/3 gap-1',
item: 'p-1 text-xs gap-1',
itemLeadingIcon: 'size-4',
itemLeadingAvatarSize: '3xs',
itemLeadingChip: 'size-4',
itemLeadingChipSize: 'sm',
itemTrailingIcon: 'size-4',
tagsItem: 'text-[10px]/3',
tagsItemDeleteIcon: 'size-3'
},
sm: {
base: 'px-2.5 py-1.5 text-xs gap-1.5',
leading: 'ps-2.5',
trailing: 'pe-2.5',
leadingIcon: 'size-4',
leadingAvatarSize: '3xs',
trailingIcon: 'size-4',
label: 'p-1.5 text-[10px]/3 gap-1.5',
item: 'p-1.5 text-xs gap-1.5',
itemLeadingIcon: 'size-4',
itemLeadingAvatarSize: '3xs',
itemLeadingChip: 'size-4',
itemLeadingChipSize: 'sm',
itemTrailingIcon: 'size-4',
tagsItem: 'text-[10px]/3',
tagsItemDeleteIcon: 'size-3'
},
md: {
base: 'px-2.5 py-1.5 text-sm gap-1.5',
leading: 'ps-2.5',
trailing: 'pe-2.5',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5',
label: 'p-1.5 text-xs gap-1.5',
item: 'p-1.5 text-sm gap-1.5',
itemLeadingIcon: 'size-5',
itemLeadingAvatarSize: '2xs',
itemLeadingChip: 'size-5',
itemLeadingChipSize: 'md',
itemTrailingIcon: 'size-5',
tagsItem: 'text-xs',
tagsItemDeleteIcon: 'size-3.5'
},
lg: {
base: 'px-3 py-2 text-sm gap-2',
leading: 'ps-3',
trailing: 'pe-3',
leadingIcon: 'size-5',
leadingAvatarSize: '2xs',
trailingIcon: 'size-5',
label: 'p-2 text-xs gap-2',
item: 'p-2 text-sm gap-2',
itemLeadingIcon: 'size-5',
itemLeadingAvatarSize: '2xs',
itemLeadingChip: 'size-5',
itemLeadingChipSize: 'md',
itemTrailingIcon: 'size-5',
tagsItem: 'text-xs',
tagsItemDeleteIcon: 'size-3.5'
},
xl: {
base: 'px-3 py-2 text-base gap-2',
leading: 'ps-3',
trailing: 'pe-3',
leadingIcon: 'size-6',
leadingAvatarSize: 'xs',
trailingIcon: 'size-6',
label: 'p-2 text-sm gap-2',
item: 'p-2 text-base gap-2',
itemLeadingIcon: 'size-6',
itemLeadingAvatarSize: 'xs',
itemLeadingChip: 'size-6',
itemLeadingChipSize: 'lg',
itemTrailingIcon: 'size-6',
tagsItem: 'text-sm',
tagsItemDeleteIcon: 'size-4'
}
},
variant: {
outline: 'text-highlighted bg-default ring ring-inset ring-accented',
soft: 'text-highlighted bg-elevated/50 hover:bg-elevated focus:bg-elevated disabled:bg-elevated/50',
subtle: 'text-highlighted bg-elevated ring ring-inset ring-accented',
ghost: 'text-highlighted bg-transparent hover:bg-elevated focus:bg-elevated disabled:bg-transparent dark:disabled:bg-transparent',
none: 'text-highlighted bg-transparent'
},
color: {
primary: '',
secondary: '',
success: '',
info: '',
warning: '',
error: '',
neutral: ''
},
leading: {
true: ''
},
trailing: {
true: ''
},
loading: {
true: ''
},
highlight: {
true: ''
},
type: {
file: 'file:me-1.5 file:font-medium file:text-muted file:outline-none'
},
multiple: {
true: {
root: 'flex-wrap',
tagsInput: 'border-0 bg-transparent placeholder:text-dimmed focus:outline-none disabled:cursor-not-allowed disabled:opacity-75'
},
false: {
base: 'w-full border-0 placeholder:text-dimmed focus:outline-none disabled:cursor-not-allowed disabled:opacity-75'
}
}
},
compoundVariants: [
{
color: 'primary',
multiple: true,
variant: [
'outline',
'subtle'
],
class: 'has-focus-visible:ring-2 has-focus-visible:ring-primary'
},
{
color: 'neutral',
multiple: true,
variant: [
'outline',
'subtle'
],
class: 'has-focus-visible:ring-2 has-focus-visible:ring-inverted'
},
{
color: 'primary',
variant: [
'outline',
'subtle'
],
class: 'focus-visible:ring-2 focus-visible:ring-inset focus-visible:ring-primary'
},
{
color: 'primary',
highlight: true,
class: 'ring ring-inset ring-primary'
},
{
color: 'neutral',
variant: [
'outline',
'subtle'
],
class: 'focus-visible:ring-2 focus-visible:ring-inset focus-visible:ring-inverted'
},
{
color: 'neutral',
highlight: true,
class: 'ring ring-inset ring-inverted'
},
{
leading: true,
size: 'xs',
class: 'ps-7'
},
{
leading: true,
size: 'sm',
class: 'ps-8'
},
{
leading: true,
size: 'md',
class: 'ps-9'
},
{
leading: true,
size: 'lg',
class: 'ps-10'
},
{
leading: true,
size: 'xl',
class: 'ps-11'
},
{
trailing: true,
size: 'xs',
class: 'pe-7'
},
{
trailing: true,
size: 'sm',
class: 'pe-8'
},
{
trailing: true,
size: 'md',
class: 'pe-9'
},
{
trailing: true,
size: 'lg',
class: 'pe-10'
},
{
trailing: true,
size: 'xl',
class: 'pe-11'
},
{
loading: true,
leading: true,
class: {
leadingIcon: 'animate-spin'
}
},
{
loading: true,
leading: false,
trailing: true,
class: {
trailingIcon: 'animate-spin'
}
}
],
defaultVariants: {
size: 'md',
color: 'primary',
variant: 'outline'
}
}
}
})
]
})