Chip
表示数值或状态的指示器。
用法
使用 Chip 包裹任何组件以显示指示器。
<template>
<UChip>
<UButton icon="i-lucide-mail" color="neutral" variant="subtle" />
</UChip>
</template>
颜色
使用 color
属性改变 Chip 的颜色。
<template>
<UChip color="neutral">
<UButton icon="i-lucide-mail" color="neutral" variant="subtle" />
</UChip>
</template>
尺寸
使用 size
属性改变 Chip 的尺寸。
<template>
<UChip size="3xl">
<UButton icon="i-lucide-mail" color="neutral" variant="subtle" />
</UChip>
</template>
文本
使用 text
属性设置 Chip 的文本。
5
<template>
<UChip :text="5" size="3xl">
<UButton icon="i-lucide-mail" color="neutral" variant="subtle" />
</UChip>
</template>
位置
使用 position
属性改变 Chip 的位置。
<template>
<UChip position="bottom-left">
<UButton icon="i-lucide-mail" color="neutral" variant="subtle" />
</UChip>
</template>
内嵌
使用 inset
属性将 Chip 显示在组件内部。这对于处理圆角组件很有用。
<template>
<UChip inset>
<UAvatar src="https://github.com/benjamincanac.png" />
</UChip>
</template>
独立显示
与 inset
属性一起使用 standalone
属性可以将 Chip 行内显示。
<template>
<UChip standalone inset />
</template>
示例
控制可见性
你可以使用 show
属性控制 Chip 的可见性。
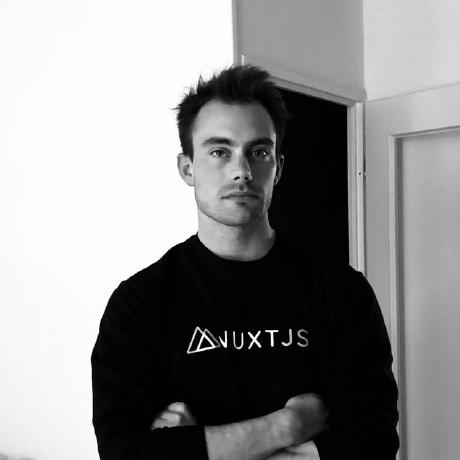
<script setup lang="ts">
const statuses = ['online', 'away', 'busy', 'offline']
const status = ref(statuses[0])
const color = computed(() => status.value ? { online: 'success', away: 'warning', busy: 'error', offline: 'neutral' }[status.value] as any : 'online')
const show = computed(() => status.value !== 'offline')
// Note: This is for demonstration purposes only. Don't do this at home.
onMounted(() => {
setInterval(() => {
if (status.value) {
status.value = statuses[(statuses.indexOf(status.value) + 1) % statuses.length]
}
}, 1000)
})
</script>
<template>
<UChip :color="color" :show="show" inset>
<UAvatar src="https://github.com/benjamincanac.png" />
</UChip>
</template>
在此示例中,Chip 根据状态显示不同的颜色,并且当状态不是
offline
时显示。API
属性
属性 | 默认值 | 类型 |
---|---|---|
as |
|
此组件应渲染为的元素或组件。 |
text |
在 Chip 内部显示一些文本。 | |
color |
|
|
size |
|
|
position |
|
Chip 的位置。 |
inset |
|
当 |
standalone |
|
当 |
show |
|
|
ui |
|
插槽
插槽 | 类型 |
---|---|
default |
|
content |
|
主题
app.config.ts
export default defineAppConfig({
ui: {
chip: {
slots: {
root: 'relative inline-flex items-center justify-center shrink-0',
base: 'rounded-full ring ring-bg flex items-center justify-center text-inverted font-medium whitespace-nowrap'
},
variants: {
color: {
primary: 'bg-primary',
secondary: 'bg-secondary',
success: 'bg-success',
info: 'bg-info',
warning: 'bg-warning',
error: 'bg-error',
neutral: 'bg-inverted'
},
size: {
'3xs': 'h-[4px] min-w-[4px] text-[4px]',
'2xs': 'h-[5px] min-w-[5px] text-[5px]',
xs: 'h-[6px] min-w-[6px] text-[6px]',
sm: 'h-[7px] min-w-[7px] text-[7px]',
md: 'h-[8px] min-w-[8px] text-[8px]',
lg: 'h-[9px] min-w-[9px] text-[9px]',
xl: 'h-[10px] min-w-[10px] text-[10px]',
'2xl': 'h-[11px] min-w-[11px] text-[11px]',
'3xl': 'h-[12px] min-w-[12px] text-[12px]'
},
position: {
'top-right': 'top-0 right-0',
'bottom-right': 'bottom-0 right-0',
'top-left': 'top-0 left-0',
'bottom-left': 'bottom-0 left-0'
},
inset: {
false: ''
},
standalone: {
false: 'absolute'
}
},
compoundVariants: [
{
position: 'top-right',
inset: false,
class: '-translate-y-1/2 translate-x-1/2 transform'
},
{
position: 'bottom-right',
inset: false,
class: 'translate-y-1/2 translate-x-1/2 transform'
},
{
position: 'top-left',
inset: false,
class: '-translate-y-1/2 -translate-x-1/2 transform'
},
{
position: 'bottom-left',
inset: false,
class: 'translate-y-1/2 -translate-x-1/2 transform'
}
],
defaultVariants: {
size: 'md',
color: 'primary',
position: 'top-right'
}
}
}
})
vite.config.ts
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import ui from '@nuxt/ui/vite'
export default defineConfig({
plugins: [
vue(),
ui({
ui: {
chip: {
slots: {
root: 'relative inline-flex items-center justify-center shrink-0',
base: 'rounded-full ring ring-bg flex items-center justify-center text-inverted font-medium whitespace-nowrap'
},
variants: {
color: {
primary: 'bg-primary',
secondary: 'bg-secondary',
success: 'bg-success',
info: 'bg-info',
warning: 'bg-warning',
error: 'bg-error',
neutral: 'bg-inverted'
},
size: {
'3xs': 'h-[4px] min-w-[4px] text-[4px]',
'2xs': 'h-[5px] min-w-[5px] text-[5px]',
xs: 'h-[6px] min-w-[6px] text-[6px]',
sm: 'h-[7px] min-w-[7px] text-[7px]',
md: 'h-[8px] min-w-[8px] text-[8px]',
lg: 'h-[9px] min-w-[9px] text-[9px]',
xl: 'h-[10px] min-w-[10px] text-[10px]',
'2xl': 'h-[11px] min-w-[11px] text-[11px]',
'3xl': 'h-[12px] min-w-[12px] text-[12px]'
},
position: {
'top-right': 'top-0 right-0',
'bottom-right': 'bottom-0 right-0',
'top-left': 'top-0 left-0',
'bottom-left': 'bottom-0 left-0'
},
inset: {
false: ''
},
standalone: {
false: 'absolute'
}
},
compoundVariants: [
{
position: 'top-right',
inset: false,
class: '-translate-y-1/2 translate-x-1/2 transform'
},
{
position: 'bottom-right',
inset: false,
class: 'translate-y-1/2 translate-x-1/2 transform'
},
{
position: 'top-left',
inset: false,
class: '-translate-y-1/2 -translate-x-1/2 transform'
},
{
position: 'bottom-left',
inset: false,
class: 'translate-y-1/2 -translate-x-1/2 transform'
}
],
defaultVariants: {
size: 'md',
color: 'primary',
position: 'top-right'
}
}
}
})
]
})
vite.config.ts
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import uiPro from '@nuxt/ui-pro/vite'
export default defineConfig({
plugins: [
vue(),
uiPro({
ui: {
chip: {
slots: {
root: 'relative inline-flex items-center justify-center shrink-0',
base: 'rounded-full ring ring-bg flex items-center justify-center text-inverted font-medium whitespace-nowrap'
},
variants: {
color: {
primary: 'bg-primary',
secondary: 'bg-secondary',
success: 'bg-success',
info: 'bg-info',
warning: 'bg-warning',
error: 'bg-error',
neutral: 'bg-inverted'
},
size: {
'3xs': 'h-[4px] min-w-[4px] text-[4px]',
'2xs': 'h-[5px] min-w-[5px] text-[5px]',
xs: 'h-[6px] min-w-[6px] text-[6px]',
sm: 'h-[7px] min-w-[7px] text-[7px]',
md: 'h-[8px] min-w-[8px] text-[8px]',
lg: 'h-[9px] min-w-[9px] text-[9px]',
xl: 'h-[10px] min-w-[10px] text-[10px]',
'2xl': 'h-[11px] min-w-[11px] text-[11px]',
'3xl': 'h-[12px] min-w-[12px] text-[12px]'
},
position: {
'top-right': 'top-0 right-0',
'bottom-right': 'bottom-0 right-0',
'top-left': 'top-0 left-0',
'bottom-left': 'bottom-0 left-0'
},
inset: {
false: ''
},
standalone: {
false: 'absolute'
}
},
compoundVariants: [
{
position: 'top-right',
inset: false,
class: '-translate-y-1/2 translate-x-1/2 transform'
},
{
position: 'bottom-right',
inset: false,
class: 'translate-y-1/2 translate-x-1/2 transform'
},
{
position: 'top-left',
inset: false,
class: '-translate-y-1/2 -translate-x-1/2 transform'
},
{
position: 'bottom-left',
inset: false,
class: 'translate-y-1/2 -translate-x-1/2 transform'
}
],
defaultVariants: {
size: 'md',
color: 'primary',
position: 'top-right'
}
}
}
})
]
})